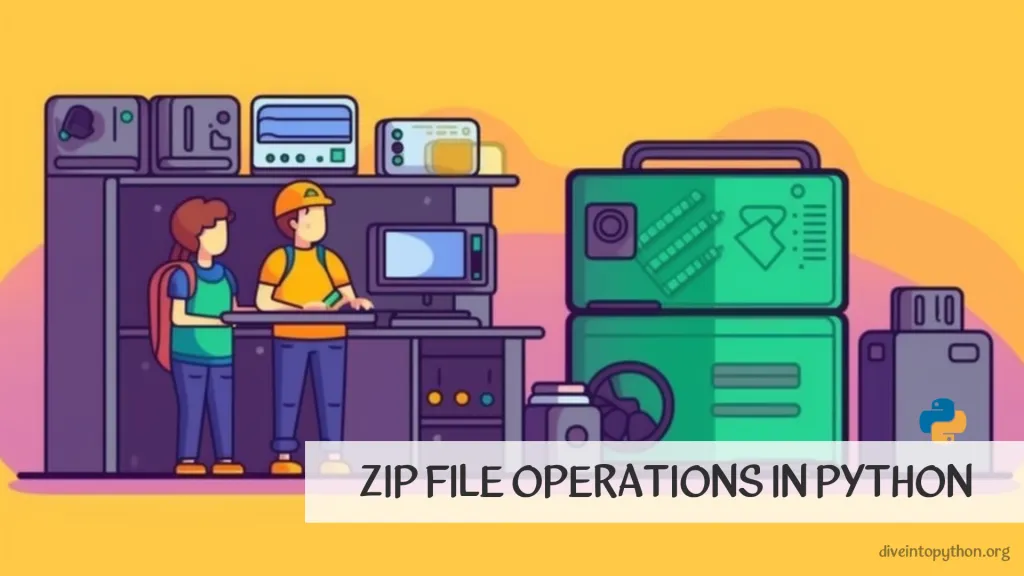
Zip 檔案是一種將多個檔案壓縮並打包成單一封存檔的熱門方式。它們通常用於檔案壓縮、資料備份和檔案分發等任務。在 Python 中壓縮或壓縮檔案是一種節省硬碟空間並讓檔案更容易透過網際網路傳輸的有用方式。
如何在 Python 中壓縮檔案
Python 中的 zipfile 模組提供建立、讀取、寫入、附加和解壓縮 ZIP 檔案的功能。
壓縮單一檔案
你可以使用 zipfile
模組建立包含單一檔案的 zip 檔案。以下是如何進行
import zipfile
# name of the new Zip file
zip_file_name = 'new_zip_file.zip'
# name of the source file
file_name = 'file_to_compress.txt'
# Create a ZipFile Object
zip_object = zipfile.ZipFile(zip_file_name, 'w')
# Add the source file to the zip file
zip_object.write(file_name, compress_type=zipfile.ZIP_DEFLATED)
# Close the Zip File
zip_object.close()
在以上程式碼中,我們首先匯入 zipfile
模組。然後我們定義 zip 檔案的名稱和來源檔案的名稱。我們建立一個 ZipFile
物件,並使用 write()
方法將來源檔案加入其中。然後我們使用 close()
方法關閉 zip 檔案。
壓縮多個檔案
你也可以建立包含多個檔案的 zip 檔案。以下是一個範例
import zipfile
# name of the new Zip file
zip_file_name = 'new_zip_file.zip'
# names of the source files
file_names = ['file_to_compressed1.txt',
'file_to_compressed2.txt',
'file_to_compressed3.txt'
]
# Create a ZipFile Object
zip_object = zipfile.ZipFile(zip_file_name, 'w')
# Add multiple files to the zip file
for file_name in file_names:
zip_object.write(file_name, compress_type=zipfile.ZIP_DEFLATED)
# Close the Zip File
zip_object.close()
在以上範例中,我們在清單中定義多個來源檔案的名稱。然後我們使用 for
迴圈和 write()
方法將這些檔案逐一加入 zip 檔案中。最後,我們使用 close()
方法關閉 zip 檔案。
若要進一步壓縮 zip 檔案,你可以將 compress_type
參數設定為 zipfile.ZIP_DEFLATED
。這會將 DEFLATE 壓縮方法套用到要壓縮的檔案。
在 Python 中解壓縮檔案
使用 zipfile
模組在 Python 中解壓縮 zip 檔案非常簡單。以下有兩種方法可以進行
import zipfile
with zipfile.ZipFile('myzipfile.zip', 'r') as zip_ref:
zip_ref.extractall('destination_folder')
在此範例中,我們首先匯入 zipfile
模組。然後我們為我們要解壓縮的 zip 檔案建立 ZipFile
類別的執行個體。r
參數表示我們要從 zip 檔案中讀取,而 myzipfile.zip
是我們要解壓縮的檔案名稱。
extractall()
方法會從 zip 檔案中擷取所有檔案,並將其儲存到指定的 destination_folder
中。如果 destination_folder
不存在,則會建立它。
import zipfile
with zipfile.ZipFile('myzipfile.zip', 'r') as zip_ref:
for file in zip_ref.namelist():
if file.endswith('.txt'):
zip_ref.extract(file, 'destination_folder')
在此範例中,我們再次匯入 zipfile
模組,並建立 ZipFile
類別的執行個體。接著,我們使用 namelist()
迴圈處理 zip 檔案中的所有檔案。如果檔案具有 .txt
副檔名,我們會將其擷取到 destination_folder
中。
透過使用這兩個程式碼範例,您可以輕鬆地使用 Python 從 zip 檔案中擷取檔案。請記得調整檔案路徑和命名,以符合您的特定需求。
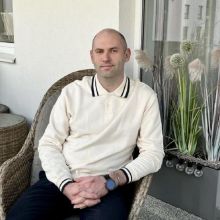
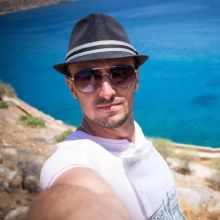